Single Threaded JavaScript: Top 5 Performance Tips

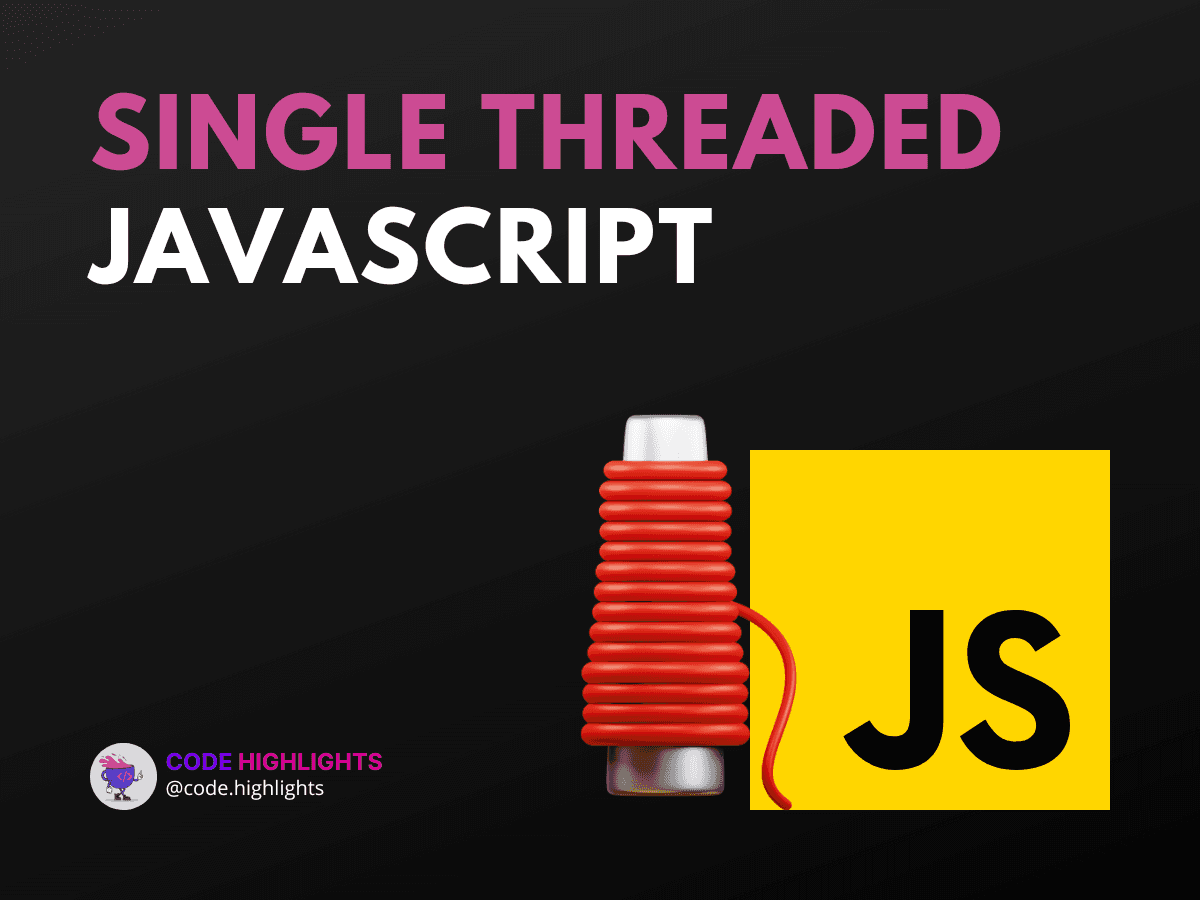
- What Does Single Threaded Mean in JavaScript?
- Is JavaScript Single Threaded Non-Blocking?
- Will JavaScript Ever Be Multithreaded?
- Is Reactjs Single Threaded?
- Conclusion
JavaScript is a powerful language that's become an essential tool for creating dynamic and interactive web experiences. However, understanding its execution model is crucial for optimizing performance, especially since it's single-threaded. Before we dive into the top performance tips, let's quickly look at a code snippet that illustrates JavaScript's syntax:
1// Simple asynchronous JavaScript example
2setTimeout(() => {
3 console.log('This message is logged after 1 second!');
4}, 1000);
In this tutorial, we'll explore how to make the most of single-threaded JavaScript, ensuring your web applications run smoothly and efficiently.
What Does Single Threaded Mean in JavaScript?
JavaScript operates on a single thread, meaning it can only execute one operation at a time. This might sound limiting, but JavaScript overcomes potential bottlenecks through non-blocking features, such as asynchronous callbacks and promises. To learn more about JavaScript and its capabilities, consider taking a course on JavaScript.
Is JavaScript Single Threaded Non-Blocking?
Yes, despite being single-threaded, JavaScript is designed to be non-blocking. It achieves this by using an event loop, which allows it to handle concurrent operations without multiple threads. Asynchronous operations, like our introductory code example, are executed later, allowing the thread to continue running other tasks.
Will JavaScript Ever Be Multithreaded?
While JavaScript itself is single-threaded, there are ways to achieve parallel processing. For instance, Web Workers allow you to run scripts in the background without affecting the main thread. However, JavaScript's core will likely remain single-threaded due to its design and how the web fundamentally works.
Is Reactjs Single Threaded?
React, a popular library for building user interfaces, also operates within JavaScript's single-threaded model. However, it introduces techniques like virtual DOM to optimize rendering and improve performance in complex applications.
Now, let's focus on the top five performance tips for single-threaded JavaScript:
-
Leverage Asynchronous Programming: Use
async/await
and promises to perform I/O-bound tasks without blocking the main thread. This allows your app to remain responsive while waiting for operations like network requests to complete. -
Optimize Loops and Iterations: Minimize work inside loops and leverage methods like
.map()
,.filter()
, and.reduce()
for array transformations. These methods can be more efficient and lead to cleaner code. -
Utilize Web Workers for Heavy Tasks: For CPU-intensive tasks, Web Workers can take the load off the main thread. This prevents UI freezes and improves user experience.
-
Efficient DOM Manipulation: Minimize direct DOM manipulation. Instead, use libraries like React or update the DOM in batches to reduce reflow and repaint costs.
-
Apply Throttling and Debouncing: These techniques are essential for optimizing events that occur frequently, such as window resizing or scrolling. Throttling limits the function execution, while debouncing ensures it only runs after a certain amount of idle time.
For a deeper dive into web development and how these languages interact, check out this course on introduction to web development.
To further your understanding of web technologies, including how HTML and CSS play a role, consider these courses on HTML fundamentals and an introduction to CSS.
Conclusion
Understanding the single-threaded nature of JavaScript is key to writing high-performance applications. By following the tips provided and using non-blocking features effectively, you can ensure your JavaScript applications are fast, responsive, and scalable.
For more insights and best practices, don't hesitate to explore external resources from reputable sources, such as Mozilla Developer Network (MDN), W3Schools, and JavaScript.info. These websites offer a wealth of information that can help you master single-threaded JavaScript and its intricacies.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
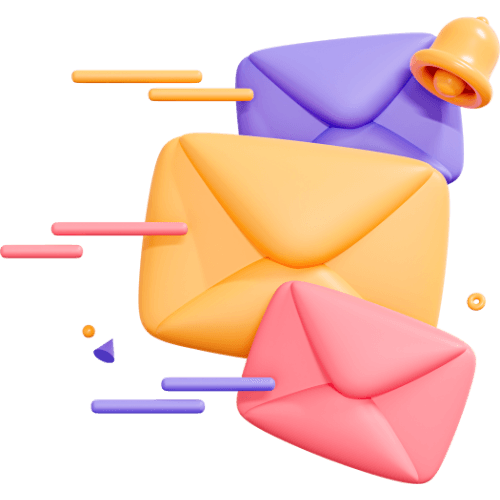
Related articles
9 Articles
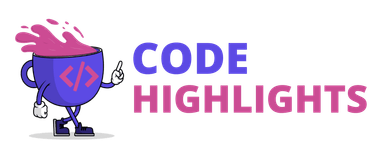
Copyright © Code Highlights 2025.