Avoid Coding Errors With Snake Case JavaScript Tactics

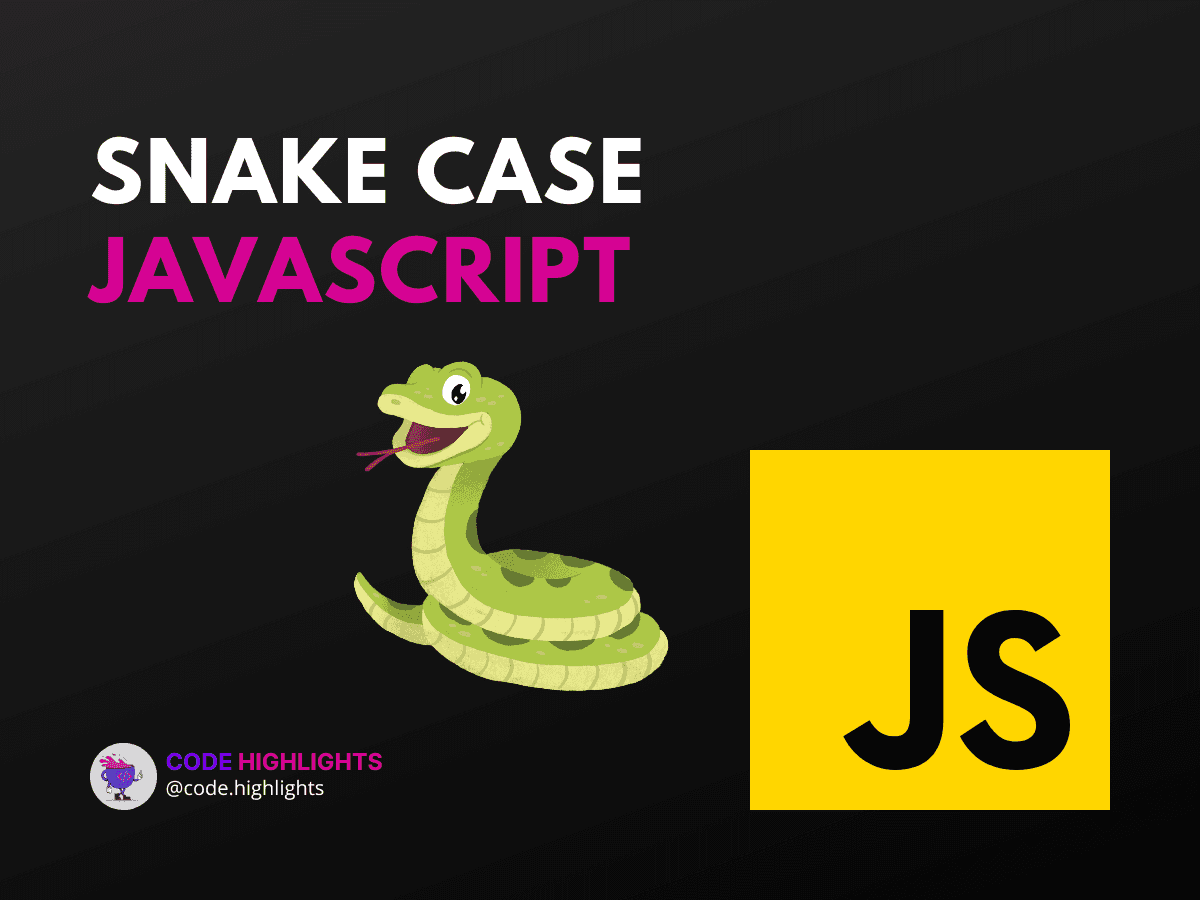
- Should I Use Snake Case in JavaScript?
- Is Snake Case Better Than CamelCase?
- How to Convert a String to Snake Case in JavaScript
- Step 1: Understand the Task
- Step 2: Create a Function
- Step 3: Use Regular Expressions
- Step 4: Join with Underscores
- Step 5: Convert to Lowercase
- Does JS Use CamelCase?
- Conclusion
JavaScript is a versatile language, powering the dynamic behavior on most websites. As you dive into the world of coding, you'll encounter various naming conventions—one of which is snake case. But what exactly is snake case, and how does it fit into JavaScript?
Before we delve into the specifics, let's quickly glimpse at JavaScript syntax with this introductory code snippet:
In the example above, my_variable_name
is written in snake case—a style where words are all lowercase and separated by underscores. Now, let's unravel the mystery of snake case in JavaScript.
Should I Use Snake Case in JavaScript?
JavaScript traditionally uses CamelCase for variable and function names. However, snake case can be useful, especially when working with JSON objects from APIs or when naming certain elements that require underscores for clarity or convention.
Is Snake Case Better Than CamelCase?
It's not about better or worse; it's about context. While CamelCase is standard in JavaScript, snake case has its place. It's prevalent in other programming environments and can enhance readability in certain scenarios. Choosing between them often comes down to team standards and the specific use case.
How to Convert a String to Snake Case in JavaScript
Converting strings to snake case in JavaScript is straightforward. Here's a step-by-step guide:
Step 1: Understand the Task
To convert a string to snake case, you'll need to identify capital letters, spaces, or other characters and replace them with an underscore followed by the lowercase version of the character.
Step 2: Create a Function
Craft a function that takes a string as input and processes it to output a snake case version.
Step 3: Use Regular Expressions
The function above uses a regular expression (/\W+/
) to split the string at any non-word character. This is a crucial step in formatting our string correctly.
Step 4: Join with Underscores
Once split, .join('_')
takes our array of words and concatenates them using underscores.
Step 5: Convert to Lowercase
Finally, .toLowerCase()
ensures all letters are in lowercase, adhering to snake case convention.
Does JS Use CamelCase?
Yes, JavaScript predominantly uses CamelCase for identifiers. But understanding and utilizing snake case can be invaluable, especially when interfacing with other systems or languages where snake case is the norm.
Conclusion
Mastering snake case in JavaScript might not be necessary for everyday coding, but it's an excellent skill to have in your toolkit. For more on JavaScript and other web development essentials, check out our HTML fundamentals course and broaden your coding horizons.
Remember, the best way to learn is by doing. Try converting different strings to snake case and see how it works with various inputs. Happy coding!
For further reading and best practices in JavaScript, consider these resources:
- Mozilla Developer Network (MDN) Web Docs on JavaScript
- W3Schools JavaScript Tutorial
- JavaScript.info for deep dives into JavaScript concepts
By integrating these steps and resources, you're well on your way to mastering snake case in JavaScript. Keep experimenting, keep learning, and most importantly, keep coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
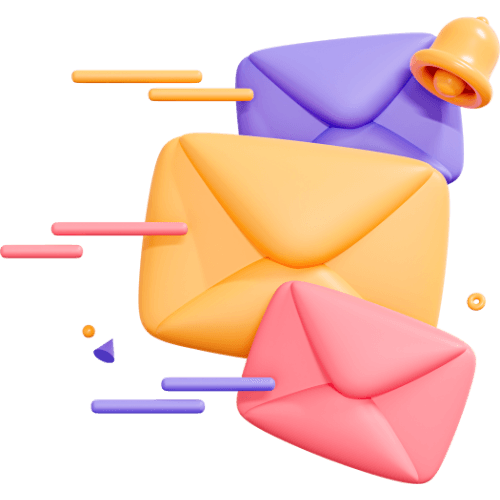
Related articles
9 Articles
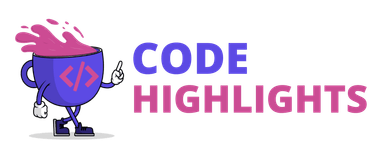
Copyright © Code Highlights 2025.