How to Sort Object by Key JavaScript for Better Performance

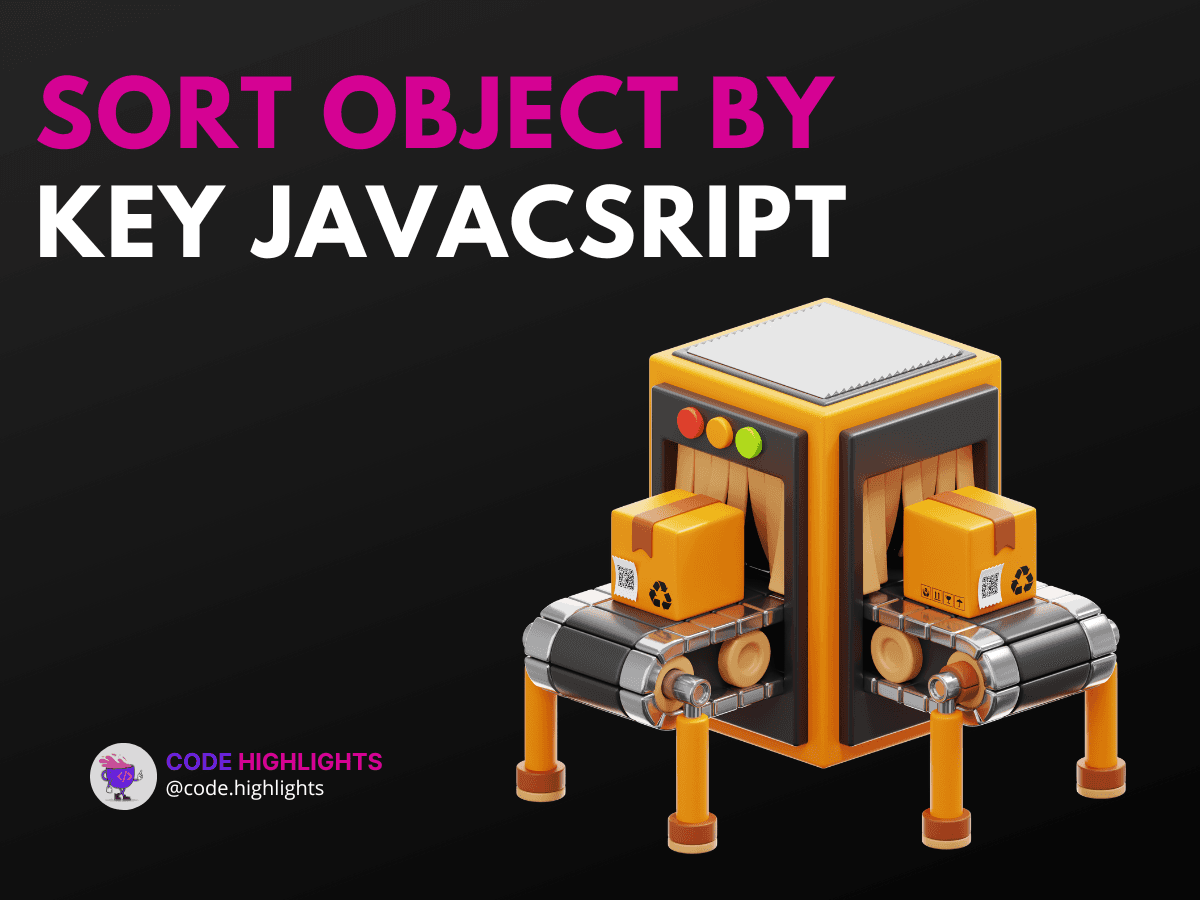
- Quick Example
- Understanding the Syntax
- Example 1: Basic Sorting of Object Keys
- Example 2: Sorting an Array of Objects by a Key
- Example 3: Sorting Nested Objects
- Compatibility with Major Web Browsers
- Summary
Sorting objects by key in JavaScript is essential for organizing data efficiently. When working with arrays of objects, you may need to sort them based on a specific property. This can enhance performance and make your code easier to understand. In this tutorial, we will explore various methods to sort an object by key in JavaScript, along with practical examples to illustrate each method.
Quick Example
Here's a quick example to get started:
1const obj = { c: 3, a: 1, b: 2 };
2const sortedKeys = Object.keys(obj).sort();
3const sortedObj = {};
4for (let key of sortedKeys) {
5 sortedObj[key] = obj[key];
6}
7console.log(sortedObj); // Output: { a: 1, b: 2, c: 3 }
Understanding the Syntax
To sort an object by key, you typically use the Object.keys()
method to retrieve an array of keys. Then, you can apply the sort()
method to sort these keys. Finally, you can create a new object based on the sorted keys.
Parameters and Return Values
- Parameters:
- The object you want to sort.
- Return Values:
- A new object sorted by the specified keys.
Example 1: Basic Sorting of Object Keys
This example demonstrates how to sort the keys of a simple object.
1const user = { name: "Alice", age: 25, city: "Wonderland" };
2const sortedUser = {};
3Object.keys(user).sort().forEach(key => {
4 sortedUser[key] = user[key];
5});
6console.log(sortedUser); // Output: { age: 25, city: "Wonderland", name: "Alice" }
Example 2: Sorting an Array of Objects by a Key
When you have an array of objects, you might want to sort them based on a specific property. Here's how you can do that:
1const users = [
2 { name: "Alice", age: 25 },
3 { name: "Bob", age: 20 },
4 { name: "Charlie", age: 30 }
5];
6
7users.sort((a, b) => a.age - b.age);
8console.log(users);
9// Output: [{ name: "Bob", age: 20 }, { name: "Alice", age: 25 }, { name: "Charlie", age: 30 }]
Example 3: Sorting Nested Objects
If you have a nested object, you can still sort it by key. Here’s an example:
1const data = {
2 user1: { name: "Alice", age: 25 },
3 user2: { name: "Bob", age: 20 },
4 user3: { name: "Charlie", age: 30 }
5};
6
7const sortedData = {};
8Object.keys(data).sort().forEach(key => {
9 sortedData[key] = data[key];
10});
11console.log(sortedData);
12// Output: { user1: { name: "Alice", age: 25 }, user2: { name: "Bob", age: 20 }, user3: { name: "Charlie", age: 30 } }
Compatibility with Major Web Browsers
Most modern web browsers support the methods used in sorting objects by key in JavaScript. You can confidently use Object.keys()
, sort()
, and forEach()
in popular browsers like Chrome, Firefox, Safari, and Edge.
Summary
In this tutorial, we learned how to sort an object by key in JavaScript. We explored different methods, including sorting simple objects, arrays of objects, and nested objects. Sorting helps improve performance and makes data handling more manageable. For more in-depth learning, consider checking out our HTML Fundamentals Course or Introduction to Web Development.
By understanding how to sort objects, you can streamline your JavaScript code and enhance its readability. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
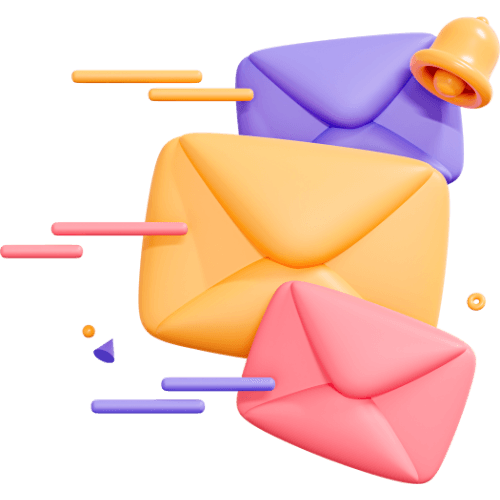
Related articles
9 Articles
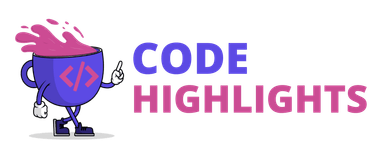
Copyright © Code Highlights 2025.