How to use the JavaScript startsWith() method

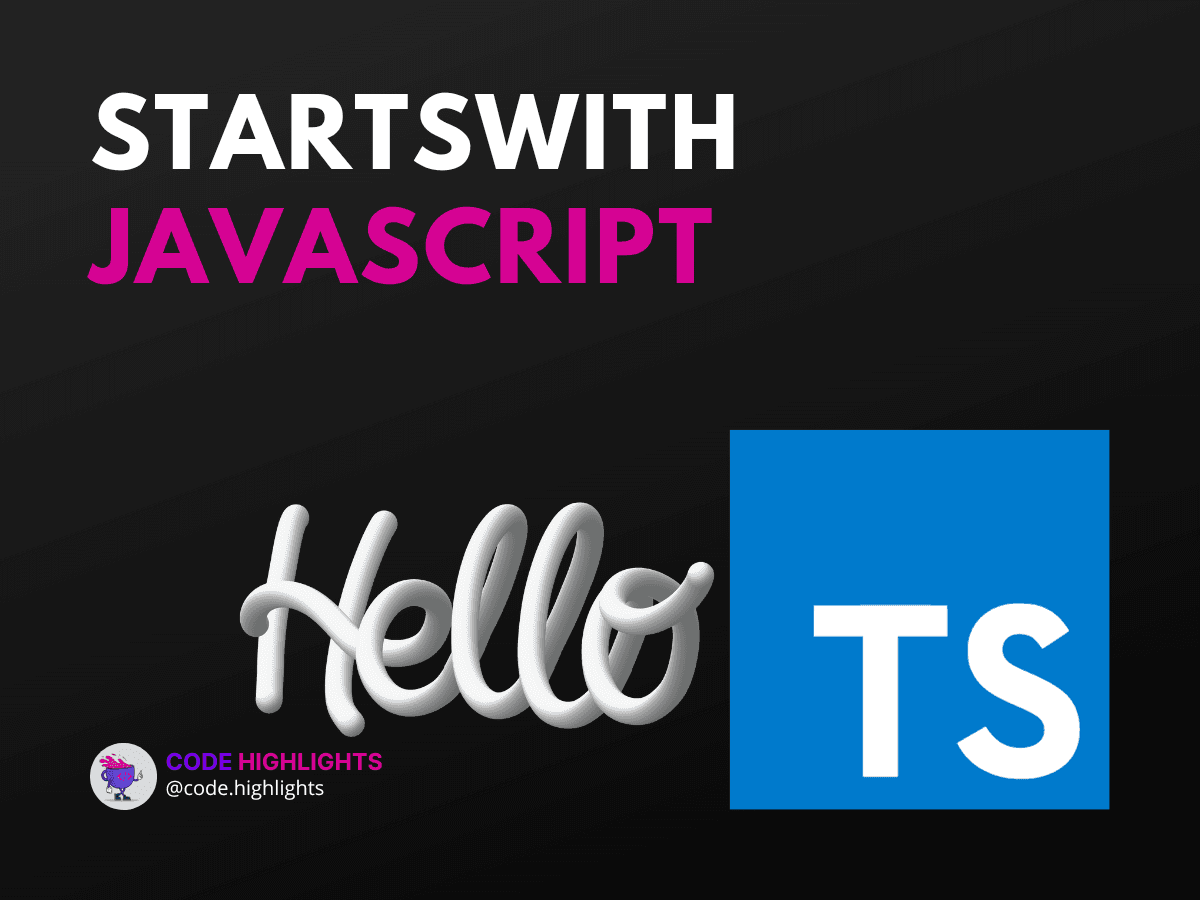
- Understanding startsWith() in JavaScript
- Step-by-Step Usage
- Practical Examples
- When to Use startsWith()
- Tips and Tricks
- Conclusion
Have you ever found yourself needing to check the beginning of a string in your JavaScript code? Whether you're validating input, searching for specific patterns, or simply organizing data, the startsWith()
method is a tool you'll want in your arsenal. In this tutorial, we'll dive into the startsWith()
method, showing you how it can be used to determine whether a string begins with certain characters.
Let's start with a quick peek at the syntax:
By the end of this guide, you'll have a solid understanding of how to use startsWith()
in your JavaScript projects. So, let's get coding!
Understanding startsWith()
in JavaScript
The startsWith()
method is a part of the String prototype in JavaScript. It allows you to check if a string starts with another substring. It's case-sensitive and straightforward to use, making your code cleaner and more readable.
Here's the method signature:
searchString
is the string you want to search for.position
(optional) is the index at which to start the search. By default, it's 0.
For example:
1let phrase = "JavaScript is fun";
2console.log(phrase.startsWith("Java")); // Output: true
3console.log(phrase.startsWith("script", 4)); // Output: true
Notice how we used the optional position
argument in the second console.log()
to specify where the method should start searching within the string.
Step-by-Step Usage
-
Identify the string: Determine the string you want to check. This will be the main string where the
startsWith()
method is applied. -
Choose the substring: Decide on the characters that you expect to be at the beginning of the main string.
-
Apply the method: Use the
startsWith()
method with the main string and the substring as arguments. -
Check the result: The method will return
true
if the main string starts with the specified characters, andfalse
otherwise.
Practical Examples
Imagine you're working on a project where you need to verify if user input begins with a specific prefix. Here's how you'd do it:
1function checkPrefix(userInput, prefix) {
2 return userInput.startsWith(prefix);
3}
4
5console.log(checkPrefix("startsWith JavaScript", "start")); // Output: true
In this function, checkPrefix
, we're checking whether userInput
starts with the prefix
provided.
When to Use startsWith()
- Validating form inputs to see if they adhere to a specific format.
- Filtering a list of strings to find items that start with a certain character or word.
- Implementing custom searching algorithms that require prefix checking.
Tips and Tricks
-
Remember,
startsWith()
is case-sensitive. If you need a case-insensitive check, convert both the main string and the substring to the same case usingtoLowerCase()
ortoUpperCase()
before callingstartsWith()
. -
The
startsWith()
method is not supported in Internet Explorer. If you need to support older browsers, consider using a polyfill or an alternative approach like a regular expression.
Conclusion
The startsWith()
method is a powerful feature in JavaScript that can save time and lines of code when dealing with strings. It's simple to use and understand, and it can be incredibly useful in various scenarios.
Now that you've learned about the startsWith()
method, you might want to expand your JavaScript knowledge by exploring our Learn JavaScript Course. If you're new to web development, don't forget to check out our Introduction to Web Development Course to build a strong foundation.
For further reading on JavaScript string methods and other related topics, check out these resources:
- Mozilla Developer Network (MDN) - String.prototype.startsWith()
- W3Schools JavaScript String Reference
- JavaScript.info - Strings
By incorporating the startsWith()
method into your JavaScript toolkit, you'll be able to write more efficient, readable, and maintainable code. Keep practicing, and soon you'll be handling strings like a pro!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
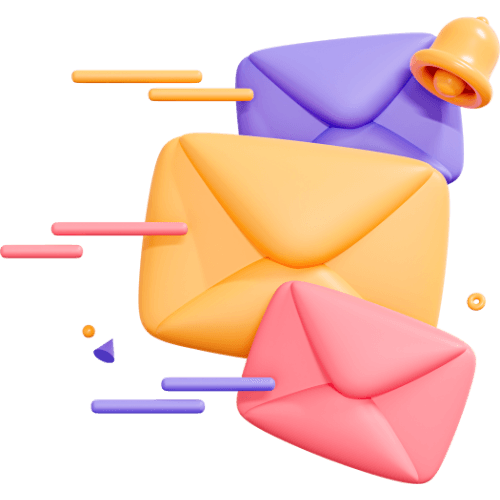
Related articles
9 Articles
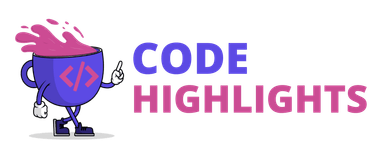
Copyright © Code Highlights 2025.