5 Tips for Mastering String Formatting in JavaScript

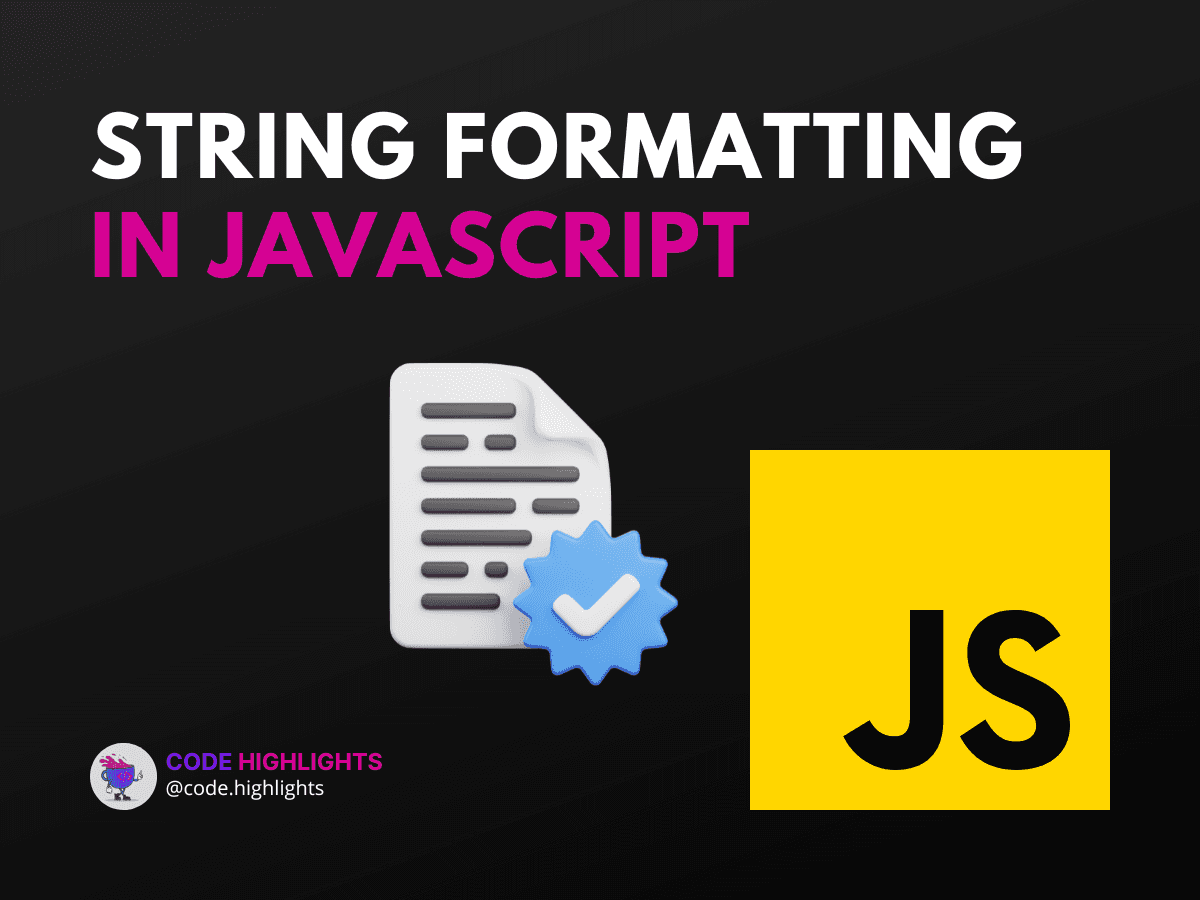
- Tip 1: Concatenation with the Plus Operator
- Tip 2: Template Literals for Easy Readability
- Tip 3: Using Template Literals for Multi-line Strings
- Tip 4: Advanced String Formatting with Intl and toLocaleString
- Tip 5: Custom Formatting Functions
String formatting is a fundamental skill in web development that can greatly enhance your JavaScript applications. Whether you're manipulating text, displaying dynamic data, or creating templates, understanding how to format strings effectively is essential. In this tutorial, we'll explore some practical tips and techniques to master string formatting in JavaScript, ensuring your code is both functional and readable.
Let's kick things off with a quick example:
Here, we've used template literals to insert the variable name
into a string. But there's much more to learn, so let's dive in!
Tip 1: Concatenation with the Plus Operator
Before ES6, string concatenation was commonly done using the plus (+
) operator:
1let firstName = "John";
2let lastName = "Doe";
3console.log("Hello, " + firstName + " " + lastName + "!");
While this method is straightforward, it can become cumbersome with complex strings. For simpler string formatting in JavaScript, consider other methods discussed below.
Tip 2: Template Literals for Easy Readability
Introduced in ES6, template literals are a game-changer for string formatting in JavaScript. They allow you to embed expressions within backticks (` `), using ${expression}
syntax:
This makes your code more readable and easier to understand compared to concatenation.
Tip 3: Using Template Literals for Multi-line Strings
Another advantage of template literals is creating multi-line strings without the need for concatenation or escaping newlines:
This keeps your HTML fundamentals clean when generating dynamic HTML content with JavaScript.
Tip 4: Advanced String Formatting with Intl and toLocaleString
For more complex formatting, such as currency, dates, or numbers, JavaScript offers the Intl
object and the toLocaleString
method. Here's an example of formatting a number as currency:
1let amount = 1234.56;
2console.log(new Intl.NumberFormat('en-US', { style: 'currency', currency: 'USD' }).format(amount));
Learning these advanced techniques is crucial for creating professional, locale-aware web applications.
Tip 5: Custom Formatting Functions
Sometimes, you might need a custom solution for string formatting in JavaScript with variables. In such cases, create a function that suits your specific needs:
1function formatGreeting(name, greeting = "Hello") {
2 return `${greeting}, ${name}!`;
3}
4console.log(formatGreeting("Mark", "Welcome"));
This approach provides flexibility and reusability across your JavaScript projects.
Remember, practice makes perfect. Experiment with these tips and incorporate them into your projects to become proficient in string formatting in JavaScript. For further learning, check out our comprehensive CSS introduction course.
To summarize, we've covered some effective strategies for string formatting in JavaScript, including the use of template literals, Intl
object, and custom functions. By mastering these techniques, you'll be able to craft dynamic and responsive web applications with ease.
For additional resources and best practices, consult reputable sources such as Mozilla Developer Network (MDN) or JavaScript.info. These platforms offer in-depth guides and documentation to further enhance your understanding of JavaScript and its capabilities.
By following these five tips, you'll be well on your way to mastering string formatting in JavaScript, making your code cleaner, more efficient, and easier to maintain. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
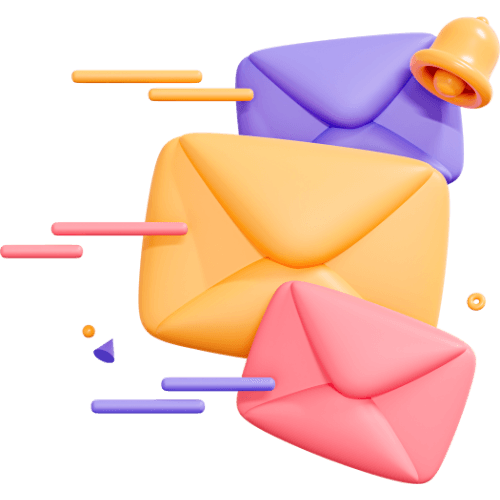
Related articles
9 Articles
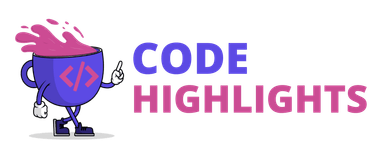
Copyright © Code Highlights 2025.