Avoid Common Pitfalls With Struct in JavaScript: Best Practices

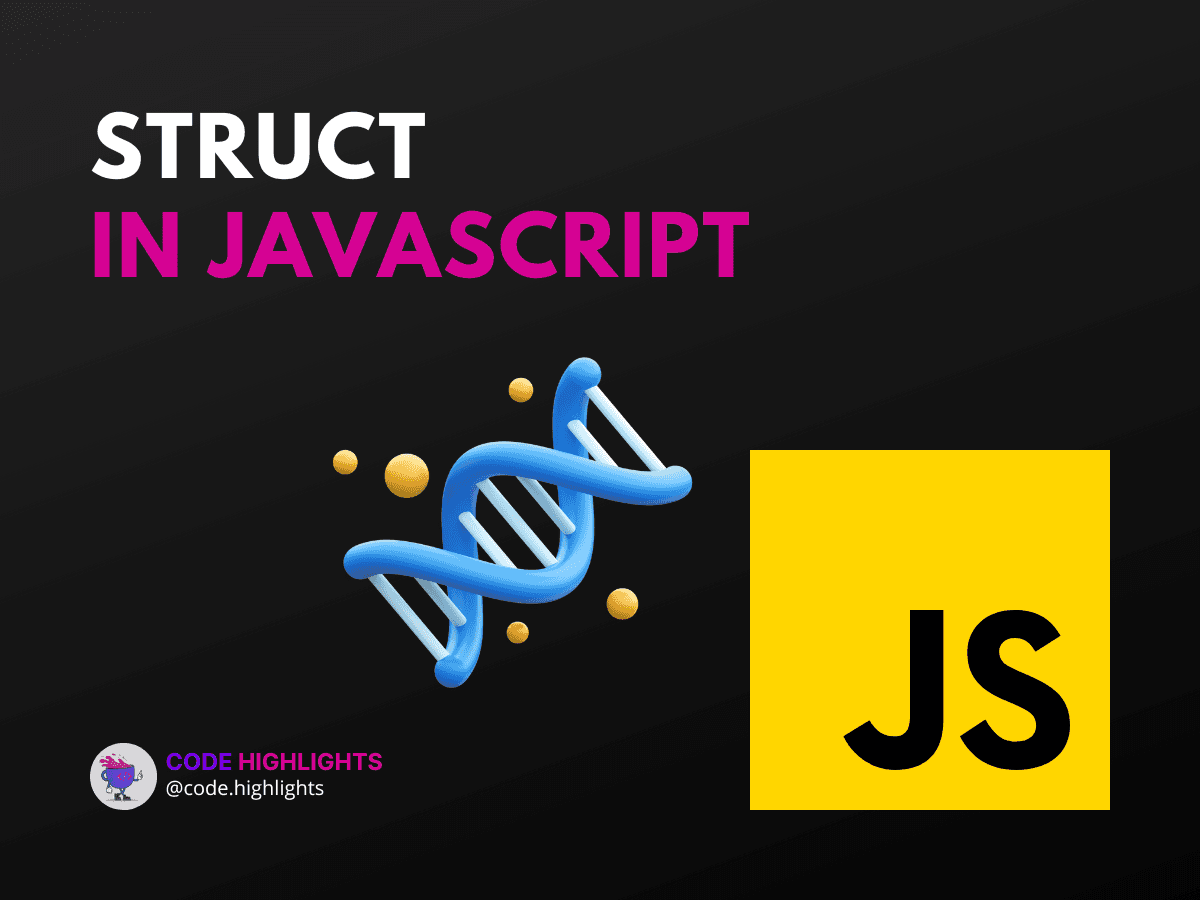
- What is a Struct in JavaScript?
- Do Structs Exist in JavaScript?
- What is the Equivalent of a Struct in JavaScript?
- What is the Difference Between Object and Struct in JavaScript?
- Best Practice #1: Use Constructor Functions or Classes
- Best Practice #2: Embrace Immutability
- Best Practice #3: Validate Property Types
- Best Practice #4: Leverage TypeScript for Type Safety
- Best Practice #5: Document with JSDoc
- Conclusion
JavaScript is a dynamic language that offers various ways to structure data. While the term "struct" may evoke thoughts of rigid, typed structures from languages like C or C++, JavaScript takes a more flexible approach. In this tutorial, we'll explore how to emulate a struct
in JavaScript, ensuring your code is both efficient and robust.
Let's start with an introductory code snippet that resembles a struct-like behavior in JavaScript:
1function Car(make, model, year) {
2 this.make = make;
3 this.model = model;
4 this.year = year;
5}
6
7const myCar = new Car('Ford', 'Mustang', 1969);
8console.log(myCar);
In the above example, we've defined a simple constructor function to create an object that holds properties similar to a struct
. But wait, what exactly is a struct in JavaScript, and do they even exist?
What is a Struct in JavaScript?
A "struct" typically refers to a custom data structure with a fixed layout of typed fields. In JavaScript, however, there are no native struct
types as seen in other languages. So, when we talk about a struct in JavaScript
, we're often referring to an object or a similar construct that serves the same purpose.
Do Structs Exist in JavaScript?
Strictly speaking, no, JavaScript does not have a struct
keyword or a direct equivalent. However, developers can mimic structs using objects, classes, or even functions with closures.
What is the Equivalent of a Struct in JavaScript?
The closest equivalent to a struct in JavaScript is an object literal or a constructor function (like our Car
example above). Objects in JavaScript are collections of properties, which can hold values of any type, making them versatile for emulating structs.
What is the Difference Between Object and Struct in JavaScript?
The main difference lies in flexibility. JavaScript objects are dynamic and can have properties added or removed at runtime. In contrast, structs in other languages are static, with a fixed set of typed fields established at compile-time.
Now that we've covered the basics, let's dive into best practices to avoid common pitfalls when attempting to use a struct in javascript
.
Best Practice #1: Use Constructor Functions or Classes
Constructor functions, as we've seen, are a great way to create template-like structures:
With the introduction of ES6, classes offer a more modern syntax:
Both approaches are effective for creating multiple instances with the same properties.
Best Practice #2: Embrace Immutability
To prevent accidental changes, consider using Object.freeze()
:
This makes your pseudo-struct immutable, avoiding unintended property reassignments.
Best Practice #3: Validate Property Types
Although JavaScript won't enforce types, you can manually check them:
Use this function to ensure the properties of your objects are of expected types.
Best Practice #4: Leverage TypeScript for Type Safety
For projects where type safety is paramount, consider using TypeScript. It extends JavaScript by adding types and interfaces, allowing you to define a struct
-like construct with strict type checking.
Best Practice #5: Document with JSDoc
Use JSDoc to document your JavaScript "structs" clearly:
1/**
2 * Represents a player.
3 * @constructor
4 * @param {string} name - The name of the player.
5 * @param {number} score - The score of the player.
6 */
7function Player(name, score) {
8 // ...
9}
Good documentation aids in maintaining struct-like constructs and clarifies their intended use.
Conclusion
While JavaScript doesn't have a built-in struct
type, you can effectively mimic this functionality using objects and best practices. Remember to structure your data thoughtfully, validate types as needed, and consider immutability to avoid common pitfalls.
If you're eager to learn more about JavaScript, consider taking a JavaScript course. For those starting out, understanding HTML fundamentals and CSS introductions is also crucial, as these technologies work hand-in-hand with JavaScript in web development. Check out this comprehensive course to get a holistic view of building web applications.
By following the guidelines we've discussed and continually refining your approach, you'll write JavaScript code that is both powerful and maintainable. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
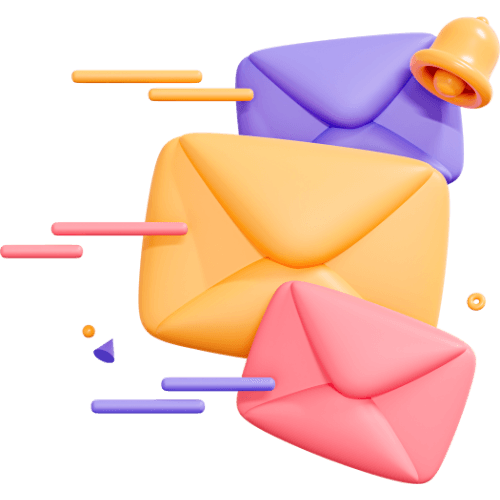
Related articles
9 Articles
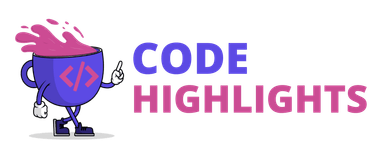
Copyright © Code Highlights 2024.