How to Use Tilde in JavaScript for Cleaner Code

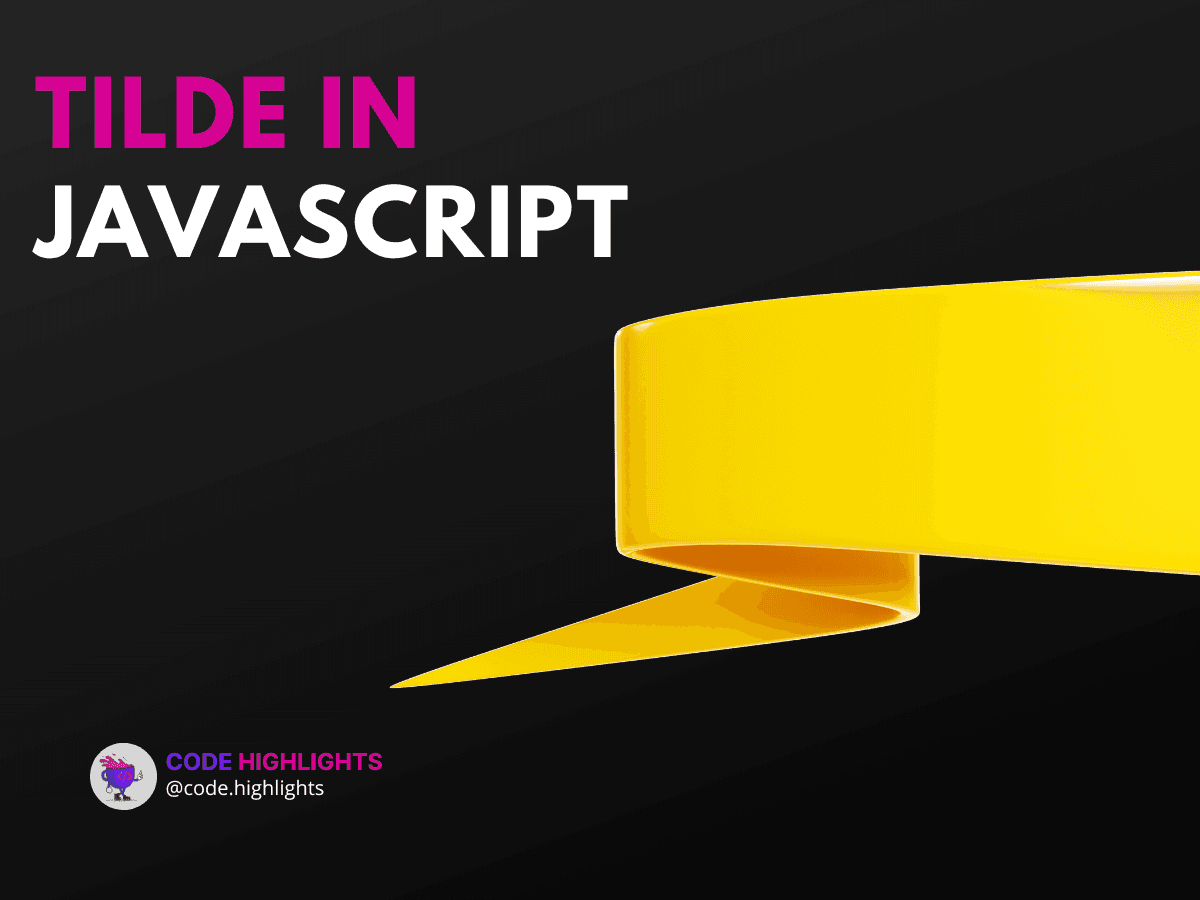
- Introduction to Tilde in JavaScript
- Syntax of the Tilde Operator
- Parameters:
- Return Value:
- Variations:
- Example 1: Tilde with Positive Numbers
- Example 2: Tilde with Negative Numbers
- Example 3: Using Tilde for Type Conversion
- Compatibility with Major Web Browsers
- Summary
JavaScript is a versatile programming language that allows developers to write efficient and clean code. One often overlooked character is the tilde (~
). This symbol can simplify your code and make it more readable. In this tutorial, we will explore how to use the tilde in JavaScript, including its syntax, variations, and practical examples.
Introduction to Tilde in JavaScript
The tilde is primarily used as a bitwise NOT operator in JavaScript. It flips the bits of a number, turning 1s into 0s and vice versa. This can be particularly useful when you want to convert a number to its negative equivalent quickly. Let's look at a simple example:
In this example, the tilde operator converts 5
into -6
.
Syntax of the Tilde Operator
The syntax for using the tilde is straightforward. Here’s how it works:
Parameters:
- value: This is a numeric value that you want to apply the tilde operator to.
Return Value:
- The operator returns the bitwise NOT of the given value.
Variations:
You can use the tilde with different types of numbers, such as integers or floating-point numbers, but remember that it operates on the integer portion.
Example 1: Tilde with Positive Numbers
Let’s see how the tilde works with positive integers:
In this case, the tilde converts 3
to -4
. This shows how the tilde can effectively negate a number.
Example 2: Tilde with Negative Numbers
The tilde also works with negative numbers:
1let negativeNum = -10;
2let negatedNegative = ~negativeNum; // negatedNegative is 9
3console.log(negatedNegative);
Here, the tilde changes -10
to 9
. This demonstrates how the operator can flip bits regardless of the sign.
Example 3: Using Tilde for Type Conversion
Another interesting use of the tilde is for converting values to integers. When you use the tilde twice (~~
), it acts like a quick way to truncate decimals:
This effectively removes the decimal part of 7.8
, resulting in 7
.
Compatibility with Major Web Browsers
The tilde operator is widely supported across all major web browsers. You can confidently use it in your JavaScript code without worrying about compatibility issues. For more details on browser compatibility, you can check out MDN Web Docs.
Summary
In this tutorial, we explored how to use the tilde in JavaScript for cleaner and more efficient code. We learned that the tilde acts as a bitwise NOT operator, flipping bits and allowing for quick negation of numbers. We also discovered how to use the double tilde (~~
) to truncate decimals easily.
Understanding the tilde in JavaScript can enhance your coding skills and help you write cleaner code. If you're interested in learning more about JavaScript or web development, consider checking out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. For a broader perspective, you can also explore our Introduction to Web Development course.
By mastering these concepts, you'll be well on your way to becoming a proficient web developer!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
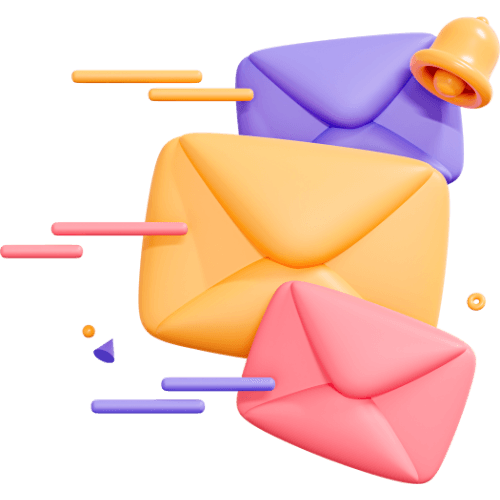
Related articles
9 Articles
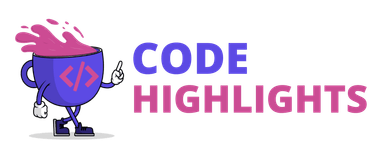
Copyright © Code Highlights 2025.