Master toLowercase JavaScript: Your Ultimate Guide for 2023!

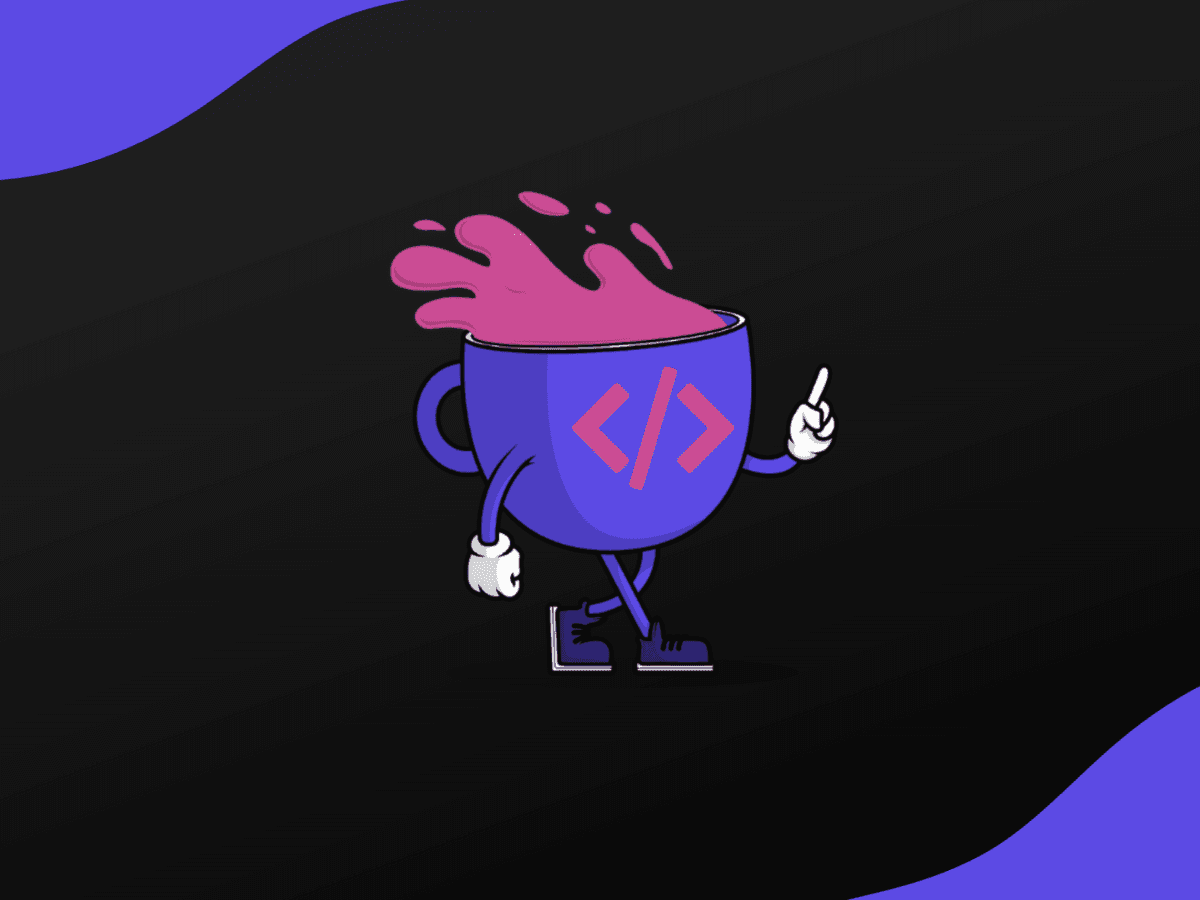
- toLowerCase()
- Using toLowerCase() with Arrays
- Case Insensitive Search
- Browser Compatibility
In JavaScript, manipulating strings is a common task. One such manipulation is converting a string to lowercase using toLowerCase()
method. This method does not affect any of the special characters, digits, and the alphabets already in lowercase.
toLowerCase()
The toLowerCase()
method in JavaScript converts all the uppercase characters in a string to lowercase characters and returns the result. It does not change the original string.
1var text = "JavaScript Tutorial";
2var result = text.toLowerCase(); // returns "javascript tutorial"
Using toLowerCase()
with Arrays
You can also use toLowerCase()
with arrays. This is particularly useful when you want to normalize data for comparison.
1var array = ["JavaScript", "HTML", "CSS"];
2var lowerCaseArray = array.map(item => item.toLowerCase());
3console.log(lowerCaseArray); // returns ["javascript", "html", "css"]
Case Insensitive Search
toLowerCase()
is very useful for case insensitive search. By converting both the source and target strings to lowercase, you can ensure that the search is case insensitive.
1var text = "Learn JavaScript";
2var searchTerm = "JAVASCRIPT";
3if (text.toLowerCase().indexOf(searchTerm.toLowerCase()) > -1) {
4 console.log("Found");
5} else {
6 console.log("Not Found");
7} // Will log `Found`
Browser Compatibility
The toLowerCase()
method is compatible with all modern browsers, and IE6 and up. If you found this post useful, you might also like our JavaScript Course.
For more in-depth understanding, you can refer to MDN's documentation on toLowerCase().
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
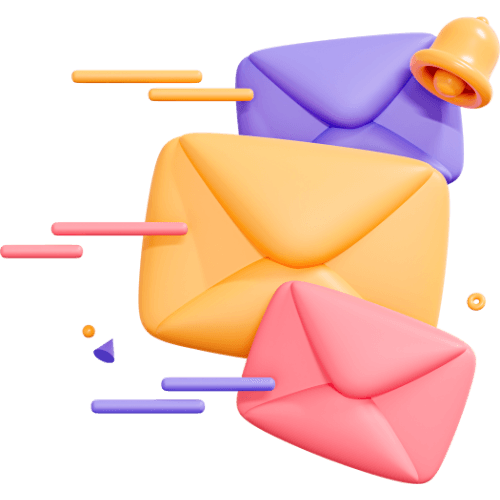
Related articles
114 Articles
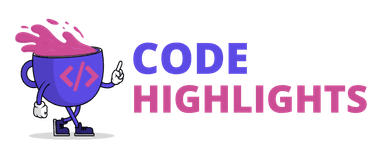
Copyright © Code Highlights 2024.