Resolve 'try catch not catching error javascript' issues. Learn how to fix it now!

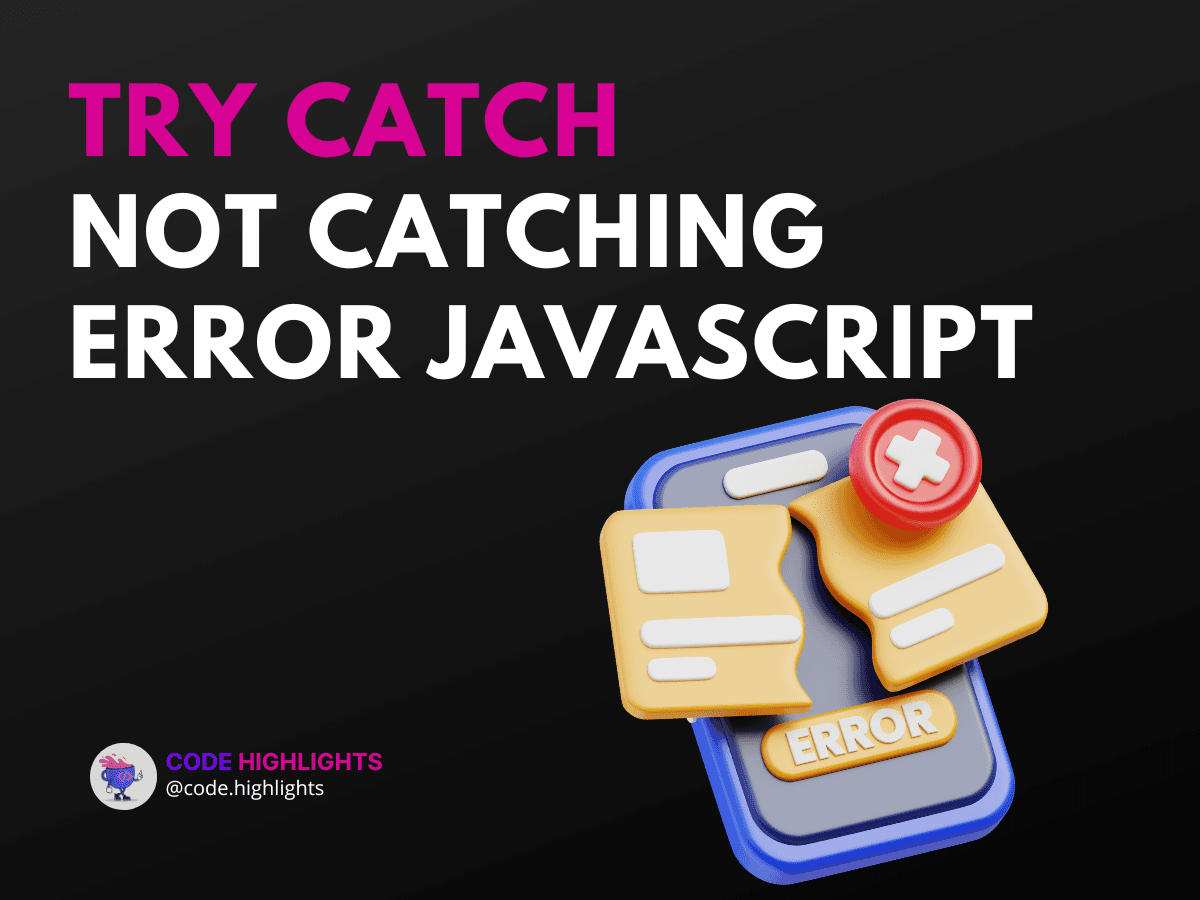
- Introduction to try...catch
- Syntax Breakdown
- Example Syntax
- Common Issues with try...catch
- 1. Synchronous vs. Asynchronous Errors
- 2. Using Promises
- 3. Throwing Errors in the Catch Block
- Compatibility with Major Browsers
- Summary
- Additional Resources
Resolving 'try catch not catching error javascript' Issues
JavaScript is a powerful programming language, but sometimes things don't go as planned. One common issue developers face is when the try...catch
statement fails to catch an error. This can be frustrating, especially when debugging your code. In this tutorial, we will explore why this happens and how to fix it effectively.
Introduction to try...catch
The try...catch
statement allows you to test a block of code for errors. If an error occurs, the control jumps to the catch
block. Here's a simple example:
1try {
2 // Code that may throw an error
3 let result = riskyFunction();
4} catch (error) {
5 console.error("Error caught:", error);
6}
In this snippet, if riskyFunction()
throws an error, it will be caught and logged.
Syntax Breakdown
The syntax of try...catch
is straightforward. It consists of two main parts:
- try: The block of code that might throw an error.
- catch: The block of code that handles the error.
Example Syntax
- Parameters: The
catch
block receives the error object. - Return Values: The
try...catch
does not return a value; it just manages errors.
Common Issues with try...catch
1. Synchronous vs. Asynchronous Errors
One reason for try catch not catching error javascript
is the difference between synchronous and asynchronous code. Errors in asynchronous code, like those from setTimeout
or promises, won't be caught by try...catch
. Here’s an example:
1try {
2 setTimeout(() => {
3 throw new Error("This error won't be caught!");
4 }, 1000);
5} catch (error) {
6 console.error("Caught an error:", error);
7}
In this case, the error won't be caught because it's thrown asynchronously. To handle it, use .catch()
with promises or the async/await
syntax.
2. Using Promises
When working with promises, ensure you handle errors properly. Here's how:
1function fetchData() {
2 return new Promise((resolve, reject) => {
3 // Simulating an error
4 reject("Error fetching data");
5 });
6}
7
8fetchData()
9 .then(data => console.log(data))
10 .catch(error => console.error("Caught an error:", error));
In this example, the error is handled in the .catch()
method.
3. Throwing Errors in the Catch Block
It's possible to throw an error within the catch
block. This can help re-throw an error for further handling:
1try {
2 throw new Error("Initial error");
3} catch (error) {
4 console.error("Caught an error:", error);
5 throw new Error("Re-thrown error");
6}
This approach can be useful for logging errors before passing them up the stack.
Compatibility with Major Browsers
The try...catch
statement is well-supported across all major browsers, including Chrome, Firefox, Safari, and Edge. However, remember that handling asynchronous errors requires different techniques.
Summary
In this tutorial, we explored the common issue of try catch not catching error javascript
. We learned that synchronous and asynchronous errors behave differently and how to handle each case effectively. Remember to use .catch()
for promises and consider re-throwing errors in the catch
block for better error management.
If you're interested in enhancing your JavaScript skills further, check out our JavaScript course. Understanding these concepts is crucial for any web developer.
For more information on web development, consider exploring our HTML Fundamentals Course and Introduction to Web Development.
Additional Resources
For a deeper understanding of error handling in JavaScript, check out these resources:
By following these guidelines, you can effectively manage errors in your JavaScript code and enhance your programming skills!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
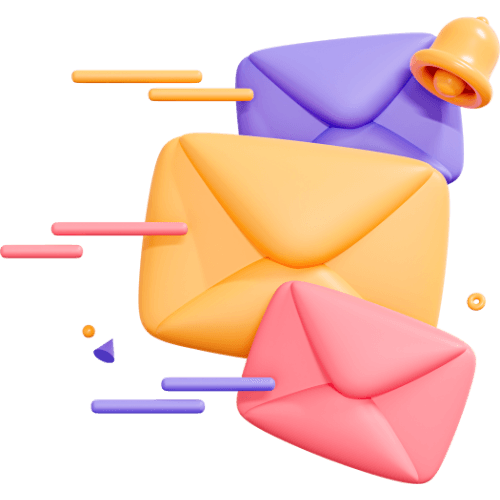
Related articles
9 Articles
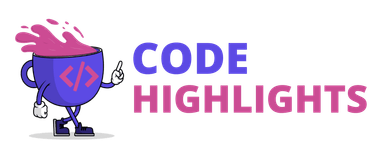
Copyright © Code Highlights 2024.