Master Unshift JavaScript: 3 Pro Tips and Hacks!

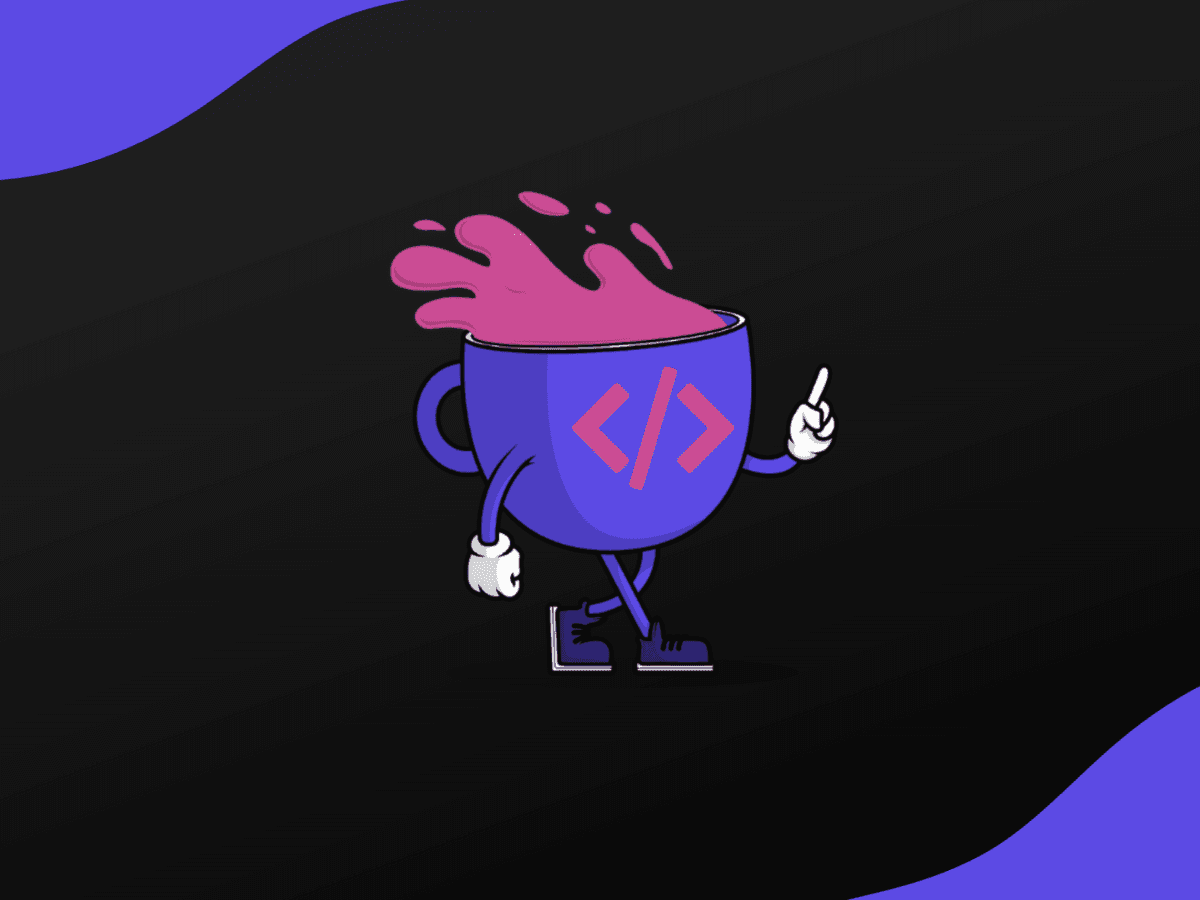
- Understanding unshift() in JavaScript
- Using shift() and unshift()
- push() vs unshift()
- reverse() vs unshift()
- Browser Compatibility
In JavaScript, arrays are used to store multiple values in a single variable. It's a complex data type that allows us to store multiple values as a single entity. There are many methods to manipulate these arrays and today, we're going to focus on the unshift()
method.
Understanding unshift()
in JavaScript
The unshift()
method in JavaScript adds new items to the beginning of an array, and returns the new length. Unlike the push()
method which appends elements to the end of an array, unshift()
prepends elements to the start.
Using shift()
and unshift()
The shift()
method works in the opposite way to unshift()
. It removes the first item from an array and returns that removed element. This method changes the length of the array.
push()
vs unshift()
While push()
adds elements to the end of an array, unshift()
adds them to the beginning. Both methods modify the original array and return the new length of the array.
1var numbers = [1, 2, 3];
2numbers.push(4); // returns 4, numbers will be [1,2,3,4]
3numbers.unshift(0); // returns 5, numbers will be [0,1,2,3,4]
reverse()
vs unshift()
The reverse()
method in JavaScript reverses the order of the elements in an array. unshift()
, on the other hand, adds one or more elements to the beginning of an array and returns the new length of the array.
1var numbers = [1, 2, 3];
2numbers.reverse(); // returns [3,2,1]
3numbers.unshift(0); // returns 4, numbers will be [0,3,2,1]
Browser Compatibility
All these methods are compatible with all modern browsers, and IE6 and up. For more information, you might find this MDN web docs useful.
You can learn more about JavaScript arrays in our JavaScript course.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
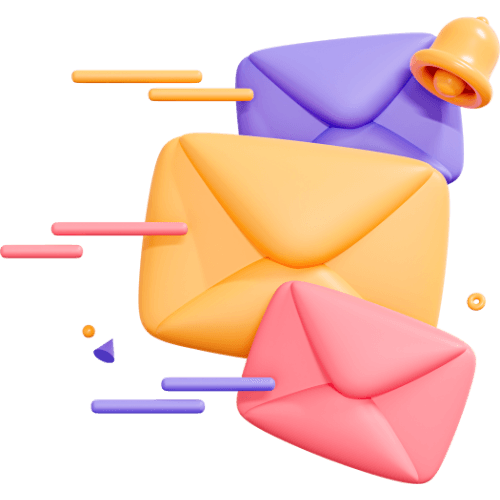
Related articles
9 Articles
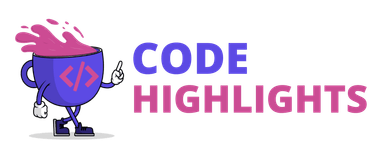
Copyright © Code Highlights 2025.