How to Use JavaScript for Web Scraping and Data Extraction

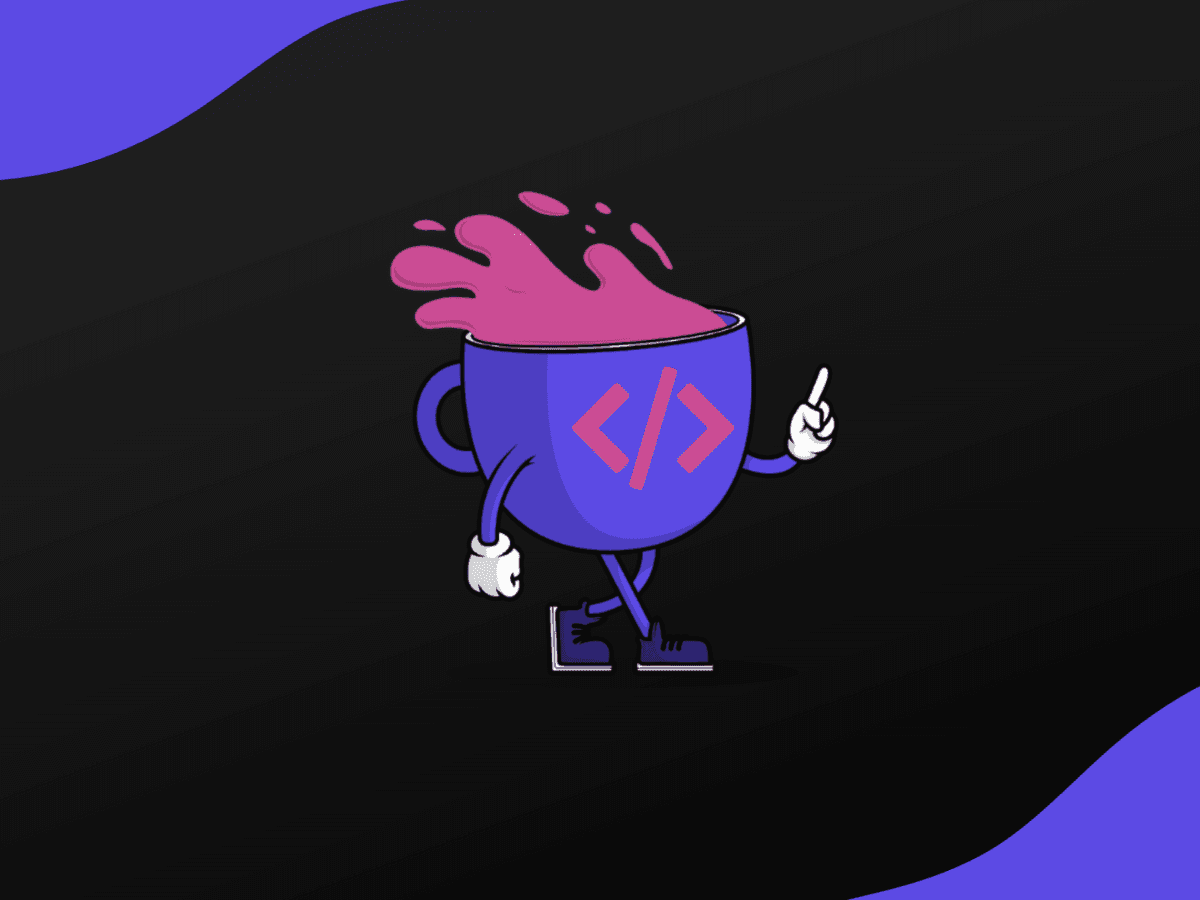
- Getting Started with Web Scraping in JavaScript
- Selecting Elements from a Webpage
- Extracting Data from Webpages
- Working with External Libraries
- Conclusion
Web scraping is the process of extracting data from websites. It has become a popular technique in fields such as data analysis and machine learning, as it enables researchers to collect large amounts of data quickly and easily. Many web scraping tools exist, but JavaScript is becoming an increasingly popular language for web scraping due to its versatility and ease of use.
Getting Started with Web Scraping in JavaScript
Before diving into web scraping in JavaScript, it is important to understand the basics of the language. JavaScript is a client-side language that is used in web development to add interactivity to websites. It is executed by the browser and can manipulate the HTML and CSS of a webpage.
To begin web scraping, you will first need to install a Node.js, a JavaScript runtime environment. Once installed, you can use Node.js to execute JavaScript scripts outside of the browser environment.
Selecting Elements from a Webpage
To extract data from a website, you will need to first select the elements you want to scrape. This can be done using CSS selectors. For example, to select all the links on a webpage, you can use the following code:
This code selects all elements with the a
tag on the webpage and stores them in an array called links
. Once you have selected the elements you want to scrape, you can loop through them to extract the information you need.
Extracting Data from Webpages
Once you have selected the elements you want to extract data from, you can use JavaScript to extract the information you need. For example, to extract all the text from a webpage, you can use the following code:
1const paragraphs = document.querySelectorAll('p');
2const texts = [];
3
4paragraphs.forEach((paragraph) => {
5 texts.push(paragraph.textContent);
6});
7
8console.log(texts);
This code selects all elements with the p
tag on the webpage and stores them in an array called paragraphs
. It then loops through each paragraph and extracts the text content using the textContent
property. The extracted text is then stored in an array called texts
, which is logged to the console.
Working with External Libraries
While JavaScript provides a lot of functionality for web scraping, there are also many external libraries that can simplify the process. For example, the puppeteer
library can be used to navigate and scrape dynamic websites.
1const puppeteer = require('puppeteer');
2
3(async () => {
4const browser = await puppeteer.launch();
5const page = await browser.newPage();
6await page.goto('https://example.com');
7
8const links = await page.$$eval('a', (links) => {
9 return links.map((link) => link.href);
10});
11
12console.log(links);
13
14await browser.close();
15})();
This code uses puppeteer
to launch a new browser page and navigate to the specified URL. It then selects all elements with the a
tag and returns an array of their href
attributes. The extracted links are then printed to the console.
Conclusion
JavaScript is a powerful language for web scraping, offering versatility and ease of use. By selecting elements on a webpage and extracting data using JavaScript, you can quickly and easily collect large amounts of data for analysis. And with the help of external libraries like puppeteer
, the web scraping process can be even more streamlined.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
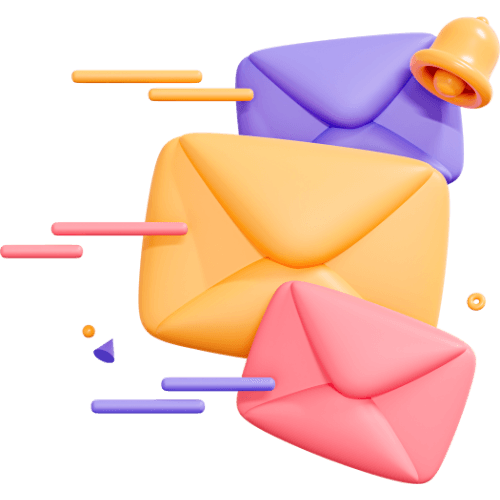
Related articles
9 Articles
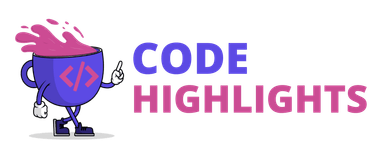
Copyright © Code Highlights 2025.