Understanding the DOM Structure

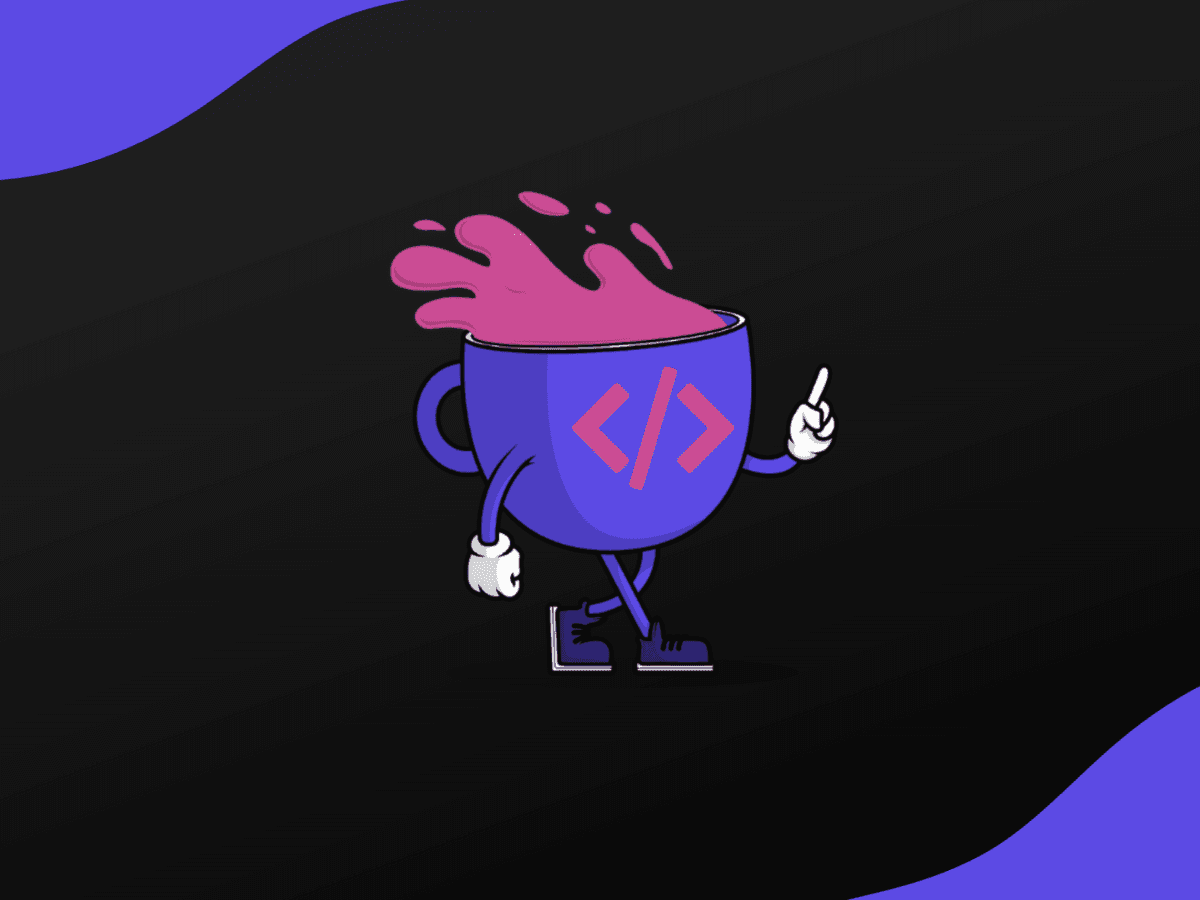
- What is the DOM?
- Why is the DOM Important in Web Development?
- How Does the DOM Work?
- Visualization of the DOM as a Tree Structure
- Nodes, Elements, and Attributes: Understanding the Components
- Relationship between HTML and the DOM
- Navigating the DOM
- Basic Navigation Methods
- Advanced Selectors using Query Selector
- Traversing Parent, Child, and Sibling Nodes
- Modifying the DOM
- Changing Element Content: textContent vs. innerHTML
- Manipulating Attributes and Classes
- Adding and Removing Elements
In today's digital landscape, websites have become increasingly sophisticated, moving from static pages to dynamic, interactive platforms. At the heart of this evolution lies a crucial component: the DOM, or Document Object Model. Whether you're a budding web developer, a seasoned programmer looking to brush up on fundamentals, or someone merely curious about the workings of a website, understanding the DOM is paramount.
What is the DOM?
In simple terms, the DOM represents the structure of a web document, typically written in HTML or XML. It's a hierarchical, tree-like representation of all the elements, attributes, and content within a page. Picture a family tree. Just as you might have grandparents, parents, and children represented in distinct lines and branches, the DOM has elements nested within elements, creating a structure that web browsers read and display.
Why is the DOM Important in Web Development?
The significance of the DOM in web development cannot be overstated. Here's why:
- Interactivity: Every interaction you experience on a website, from the simplest hover effects to complex data-driven updates, is a result of manipulating the DOM. It's the bridge between static content and dynamic user experiences.
- Programmability: The DOM provides an interface that developers can interact with using programming languages, primarily JavaScript. This allows for real-time changes on a webpage without needing to reload it. If you've ever added items to an online shopping cart or liked a social media post without being redirected to a new page, you've seen the DOM in action.
- Flexibility: Web developers can access and modify the DOM structure, style, and content dynamically. This malleability allows for the creation of responsive designs, real-time data visualization, and more. In essence, it's the backbone of modern web applications.
As we delve deeper into the intricacies of the DOM, it becomes clear that this model is not just about understanding a website's structure—it's about harnessing its potential. As the foundation of interactivity and dynamism in web development, the DOM plays a pivotal role in shaping the web as we know it.
How Does the DOM Work?
Ever wondered how a simple code on the backend translates into a dynamic, visually appealing webpage? The answer lies in the magic of the DOM. The DOM is to web developers what a canvas is to artists: a space to create, modify, and innovate. Let's dive into its mechanics.
Visualization of the DOM as a Tree Structure
The most common way to visualize the DOM is by picturing it as a ** tree structure**, aptly named the "DOM tree." At the root of this tree, we have the Document
object, representing the entire page. From there, branches sprout out, each representing various parts of the document, such as the head, body, divs, paragraphs, and so on.
Each branch, in turn, might sprout smaller branches and leaves. For instance, a branch representing a div
may have leaves representing text content, images, or even other nested divs.
By organizing the content in this hierarchical manner, browsers can quickly render a webpage and developers can efficiently navigate and manipulate the content.
Nodes, Elements, and Attributes: Understanding the Components
When delving into the DOM, three terms often crop up: nodes, elements, and attributes. Let's break them down:
- Nodes: In the DOM tree, every single part, be it the entire document, an element, an attribute, or even text content, is represented as a node. In essence, a node is a single point in the tree. The entire document is a document node, an HTML tag (like
<p>
) is an element node, and the content within that tag is a text node. - Elements: These are specific types of nodes and are the most widely interacted with. An element represents an HTML tag in the document, like
<div>
,<a>
,<p>
, and so on. Elements can have attributes and can encapsulate other elements, forming the nested tree structure. - Attributes: These are the properties that define an element's characteristics further. In the HTML tag
<img src="image.jpg" alt="A description">
, src and alt are attributes that provide additional information about the image element.
Relationship between HTML and the DOM
While they're closely related, HTML and the DOM are not the same. Think of HTML as the static blueprint of a building, detailing where each brick goes and how rooms connect. The DOM, on the other hand, is the living, breathing building constructed from that blueprint.
When a browser loads a webpage, it reads the HTML and creates the DOM based on that information. Once the DOM is constructed, it becomes the primary interaction point for scripts, even if the changes reflect in the user's view as modifications to the "HTML."
The DOM is dynamic; it updates in real-time. If you've ever interacted with a site where content changed without a page reload (like live comment feeds or interactive forms), that's the DOM in action. HTML provides the initial state, but the DOM encapsulates the current state.
Navigating the DOM
Imagine navigating through a vast forest. To find your way, you'd need a map and a compass. Similarly, in the vast forest of the DOM tree, developers need tools and methods to find specific elements and navigate the hierarchical structure. Let's delve into the toolkit of navigating the DOM.
Basic Navigation Methods
For beginners and seasoned developers alike, these basic methods serve as the starting point for most DOM interactions:
- getElementById: As the name suggests, this method retrieves an element by its unique ID. Since IDs are meant to be singular within a page, getElementById returns a single element. For example, to grab an element with the ID header, you'd use:
- getElementsByClassName: This method targets elements based on their class attribute, returning a live HTMLCollection of elements. For instance, to retrieve all elements with the class highlight, you'd use:
- getElementsByTagName: If you wish to target elements by their tag name (like div, p, or a), this method is your go-to. It also returns a live HTMLCollection. To fetch all paragraph elements:
Advanced Selectors using Query Selector
As web development evolved, so did the need for more versatile selectors. The querySelector
and querySelectorAll
methods offer more flexibility, allowing you to use CSS-like selectors:
- querySelector: Retrieves the first element that matches a specified selector. For instance, to select the first element with the class highlight:
- querySelectorAll: Fetches all elements matching the given selector, returning them as a static NodeList. For instance, to select all elements with the class highlight:
Traversing Parent, Child, and Sibling Nodes
Often, you may need to navigate relative to a specific element, moving up, down, or sideways in the DOM tree. Here's how:
- Parent Node: If you've selected an element and wish to access its immediate parent, use the parentNode property:
- Child Nodes: To access all child nodes (including non-element nodes like text nodes) of an element, use the childNodes property. For only the child elements, use children.
- Sibling Nodes: Occasionally, you might need to access elements adjacent to your selected node. For the immediately preceding sibling, use previousSibling or previousElementSibling for the element-only version. Similarly, for the next sibling, use nextSibling or nextElementSibling.
Modifying the DOM
Navigating the DOM is essential, but the real magic begins when we start modifying it. This dynamic manipulation is what powers the interactive web experiences we've come to know and love. Whether you're creating a to-do list that updates in real time, building dynamic galleries, or fashioning interactive forms, understanding how to modify the DOM is pivotal.
Changing Element Content: textContent vs. innerHTML
Before we jump into the methods, let's clear up a common question: What's the difference between textContent
and innerHTML
?
- textContent: This property represents the raw text content of an element and all its descendants. It's purely text-based, which means that it won't interpret any HTML tags as such; they'll be displayed as plain text. For example:
- innerHTML: This property gets or sets the HTML content (inner HTML) of an element. It can be used to read or replace the entire content, including the tags. For instance:
While innerHTML
provides more flexibility, it can also introduce security risks if not used judiciously, especially when injecting user-generated content. textContent
is generally safer when handling raw text.
Manipulating Attributes and Classes
Attributes like src
, href
, or id
and classes provide additional information and styling to elements. Here's how you can interact with them:
- setAttribute & getAttribute: These methods allow you to set and get attributes of an element, respectively. For instance:
- classList: This property provides a host of methods to manipulate the class list of an element. Some of its commonly used methods include:
- add(): Adds a class.
- remove(): Removes a class.
- toggle(): Toggles a class.
- contains(): Checks if a class exists.
Adding and Removing Elements
To make the web truly dynamic, we often need to add or remove elements on the fly:
- createElement: This method creates an element node. It doesn't add the element to the DOM tree but creates a node that you can append using methods like
appendChild
. - appendChild: This method adds a node to the end of the child nodes of a parent element:
- removeChild: Contrary to appendChild, this method removes a child node from a parent element:
Do note that modern browsers also support the remove()
method on the Element itself, which allows for a more straightforward element removal without referencing the parent.
The ability to modify the DOM is what breathes life into static web content. Whether you're changing text, adjusting styles, adding interactive elements, or removing outdated content, the DOM provides a robust set of tools for dynamic web manipulation.
FAQs
What is the DOM?
What is the DOM?
The DOM, or Document Object Model, is a programming interface for web documents. It represents the structure of a document as a tree of objects, where each object corresponds to a part of the document, such as elements or attributes.
How is the DOM different from HTML and CSS?
How is the DOM different from HTML and CSS?
While HTML and CSS define the structure and style of a web page, respectively, the DOM represents a live, in-memory tree-like structure of that page. It's a dynamic representation, allowing programs and scripts to modify the document's structure, style, and content in real-time.
Why is the DOM important for web developers?
Why is the DOM important for web developers?
The DOM allows scripts to dynamically access and update content, structure, and styles of a web page. It provides a structured, programmatic interface to web content, enabling rich, interactive web applications.
What is the Virtual DOM?
What is the Virtual DOM?
The Virtual DOM is a programming concept used in some modern JavaScript libraries and frameworks, like React. It's an in-memory representation of the real DOM elements. The rendering engine can quickly make changes to the Virtual DOM and subsequently update the real DOM in a more efficient and optimized manner.
How can I inspect the DOM?
How can I inspect the DOM?
You can inspect the DOM using browser developer tools. Right-click on any part of a web page and select "Inspect" or "Inspect Element." This will open the developer tools, where you can view and interact with the live DOM.
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
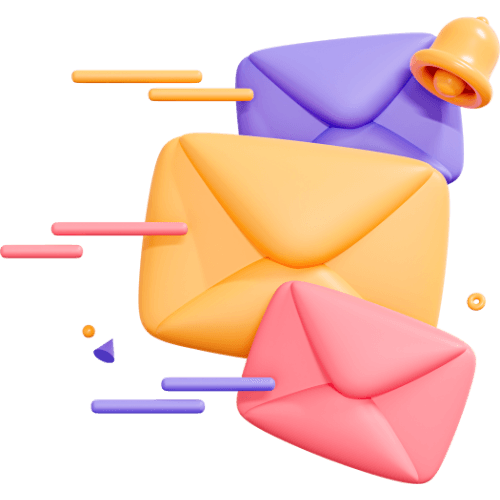
Related articles
9 Articles
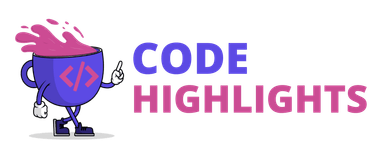
Copyright © Code Highlights 2025.