What is the Javascript alternative to the sleep function?

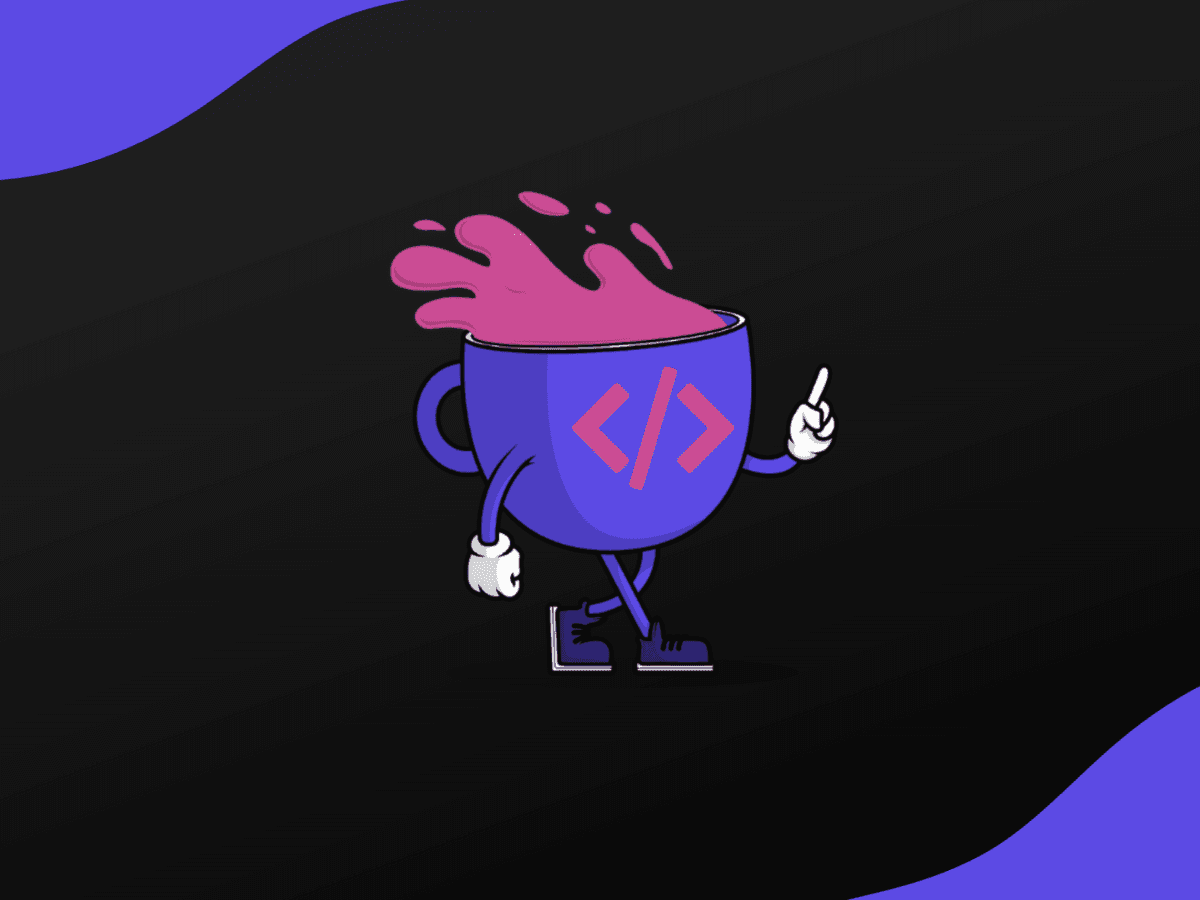
- setTimeout()
- setInterval()
- Promise with async/await
- Browser Compatibility
- Conclusion
In JavaScript, there is no in-built sleep()
function like in other languages. The sleep()
function, which pauses the execution of a program for a specified period, is a common feature in many programming languages. However, JavaScript provides alternative ways to simulate this function using setTimeout()
, setInterval()
, and Promise
with async/await
.
1// Using setTimeout
2console.log("Hello");
3setTimeout(() => {
4 console.log("World");
5}, 2000); // Waits for 2 seconds before printing "World"
setTimeout()
The setTimeout()
method allows you to delay the execution of a function by a specified number of milliseconds. It's similar to the sleep()
function found in other programming languages. The first argument is the function to execute, and the second argument is the delay time.
1var greeting = "Hello World!";
2setTimeout(function(){ alert(greeting); }, 3000); // Displays an alert box after 3 seconds
setInterval()
The setInterval()
method, like setTimeout()
, delays the execution of a function. However, it continues to call the function at the specified interval until clearInterval()
is called or the window is closed. This makes it more suitable for functions that need to be repeated at regular intervals.
1var counter = 0;
2var intervalId = setInterval(function(){
3 counter++;
4 console.log(counter);
5 if(counter === 5){
6 clearInterval(intervalId);
7 }
8}, 1000); // Increments counter every second, stops after reaching 5
Promise
with async/await
Another way to simulate a sleep function in JavaScript is by using a Promise
with async/await
. This method is especially useful in modern JavaScript development where asynchronous programming is common.
1function sleep(ms) {
2 return new Promise(resolve => setTimeout(resolve, ms));
3}
4
5async function demo() {
6 console.log('Taking a break...');
7 await sleep(2000);
8 console.log('Two seconds later');
9}
10
11demo(); // Logs "Taking a break...", waits for 2 seconds, then logs "Two seconds later"
Browser Compatibility
All three methods (setTimeout()
, setInterval()
, and Promise
with async/await
) work in all modern browsers. If you found this tutorial useful, you might also like our Learn JavaScript course. To understand how JavaScript interacts with HTML and CSS, take a look at the HTML Fundamentals and CSS Introduction courses.
Conclusion
This tutorial provides a comprehensive guide on how to simulate a sleep()
function in JavaScript using setTimeout()
, setInterval()
, and Promise
with async/await
. The use of these methods effectively mimics the functionality of the sleep()
function, allowing programmers to incorporate delays in their code execution.
Remember, while these methods can be used to simulate a sleep()
function, they are not identical to the sleep()
function found in other languages like C or Python. In JavaScript, these methods are asynchronous and non-blocking, meaning they don't stop the entire program's execution, unlike the traditional sleep()
function.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
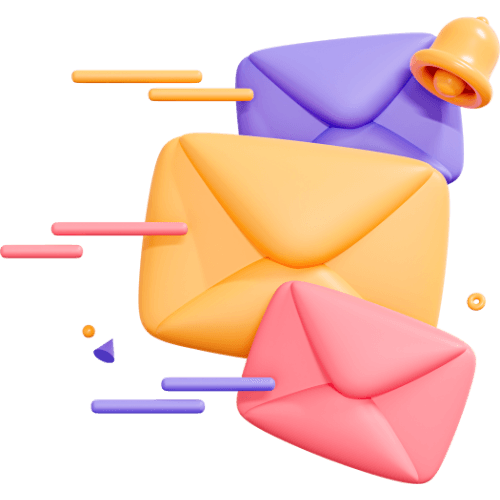
Related articles
9 Articles
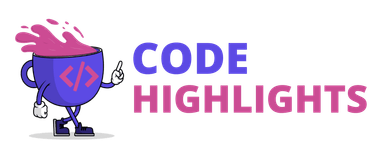
Copyright © Code Highlights 2025.